Time To Unfold Algorithms Coding Interviews
Introduction: Why am I writing this?
A practical guide that is written for those who lost their sanity trying to navigate coding interviews through competitive programming platforms!
Table of Contents:
1. Introduction
When I prepare for coding interviews, I often struggle to enjoy solving algorithm problems. This challenge is not in understanding and grasping these problems or coming up with a simple brute-force solution, but in where and how I use them in my daily work routine.
One of the reasons is that my dopamine system gets triggered when I encounter a real-world problem that affects humans. It is not just the problem, but the story behind it; who are the people affected by it? How do they get affected? Why do they get affected? Does the problem come from the perspective of “I need to do X” or “I want to do X”?
Another reason is, as an applied machine learning scientist, Approaching data problems has a systematic way of thinking. For example, you can not go to the modeling step if you do not come across the exploratory data analysis step (EDA) to understand potential problems in data. However that is not the case for algorithm problems because each problem could be solved with a different thought process, making it challenging when approaching them the first time.
Also, the nature of data science problems, by default, they are coupled with humans. Directly, like credit card frauds, or indirectly, like weld defects in oil pipelines. But that is not the case regarding data structure and algorithm-related problems or, let us say, competitive programming challenges. They come in an abstract way, with no story behind each problem or any human that got affected by them. This could make the process of tackling these types of problems a bit boring if you are a person like me!
Finally, when I study algorithms, I seek visual intuition to understand the problems and their solutions. That sometimes could be a daunting process to apply to some problems.
2. But… I do not want to waste my time
You could ask yourself…
Why do I need to solve data structure and algorithm problems when I do not need them in my daily work routine?
The answer to this question could be:
Fast Evaluation: Companies need a fast approach to test the coding skills of future candidates. (more weight in junior roles and a bit relaxed in senior roles).
Familiar with Patterns: Companies want you to be aware of the most important data structure and algorithms patterns so that you can apply them when they occur.
Standard Approach: Despite their lack of assessing all candidate skills, algorithm-based coding questions have become a standard approach to evaluating the coding skills of future candidates.
Ok, now, If you are a person like me, you may have this hectic question in your mind:
How can I enjoy practicing algorithms problems while being effective and efficient during interview preparation?
Personally, I tried different approaches to make the preparation time enjoyable, but after a back-and-forth, I found the following working recipe, which I called the FGCC method:
Focus (F): Focus on recognizing repetitive patterns when tackling algorithm problems.
Group (G): Group those problems by similar patterns.
Convert (C): Convert each pattern group to a solution template you can use if one pattern pops up again in other problems.
Communicate (C): Communicate those patterns with other people. (this is a crucial step).
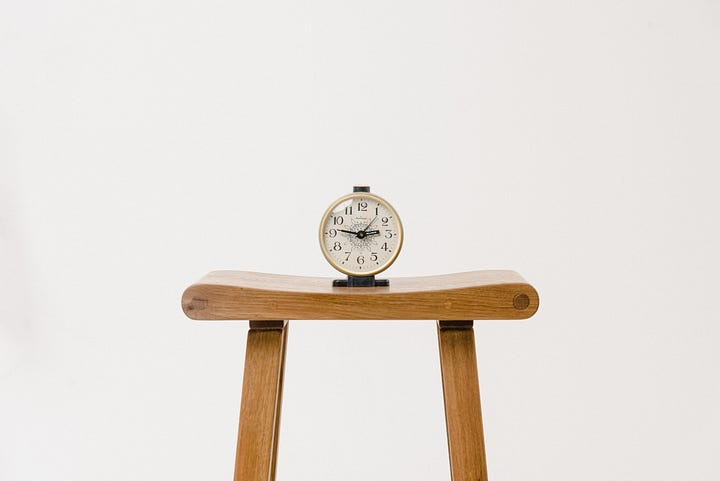
But you might say: “hmm, doing that needs time, Waleed!!”. Yes, I agree, and it is something that we fight to have every day in this busy world (i.e., your coding interview next week). Therefore, the goal of this series will be as an extensive cheatsheet to help you to:
Narrow down your focus toward important problems.
Provide you with some patterns.
Group some problems according to these patterns.
3. The Rise Of FGCC Technique: Act as a Machine Learning Algorithm
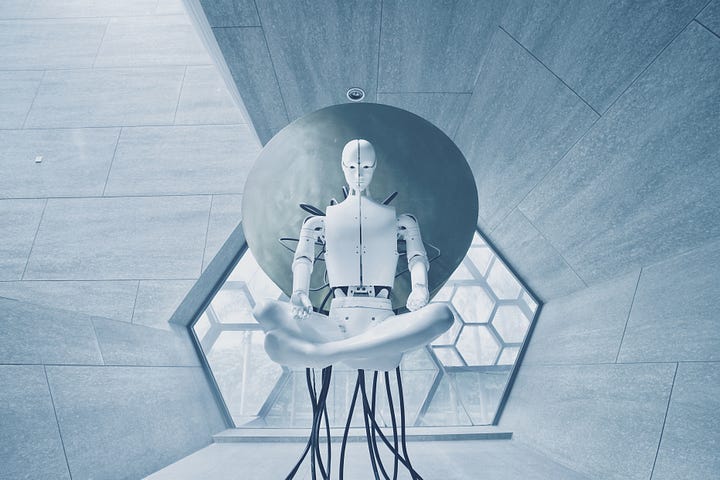
The intuitive idea of FGCC comes from my work nature as an applied machine learning scientist. That is because training machine learning (ML) algorithm with a few examples is one of the things that I have to deal with during my work. (i.e., Natural Language Processing (NLP) & BERT).
⚑ Note 1
A machine learning algorithm is (not all them ) a bunch of weights that are updated during a training process using one or more datasets.
A model is a trained machine learning algorithm.
The basic idea is that you do not have to start training your machine learning algorithm from scratch. All you need is a baseline model trained on some public datasets (i.e., articles from Wikipedia) and start the training (warm start). An example of such a baseline model is GPT-3, and a model built upon GPT-3 baseline is ChatGPT.
Ok, Waleed, what do you want to say? Alright, what I want to bring here is that we can use the same analogy in our preparation for coding interviews.
First, we need to have a mental baseline for the warm starting. This baseline is built by reviewing other people’s solutions but to some algorithm problems. Second, we need to develop our tailored model to solve any algorithm problem that could pop up during any interview we conduct.
Focus (F) and Group (G) are responsible for building this mental baseline, while Convert (C), and Communicate (C) produce the tailored baseline. In the roadmap section, I will tell you how we practically do this!
4. To Whom Is This Series?
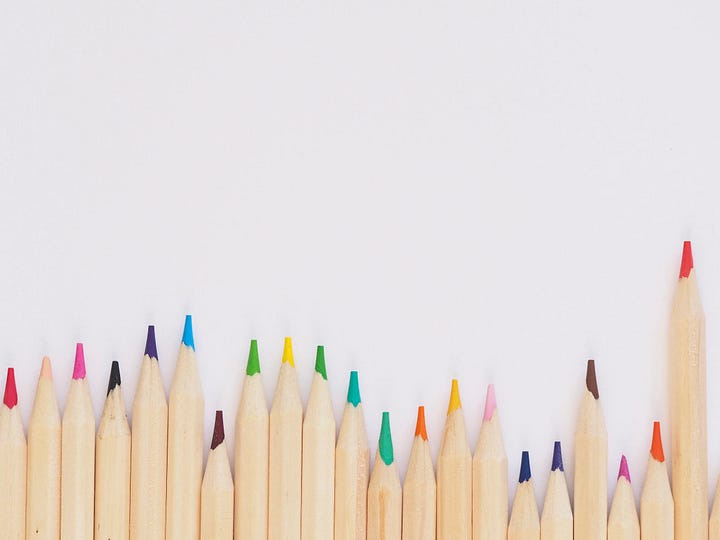
This series is for rainy days, and it is well-tailored for you if you:
Have a coding interview, and you rush to cover as many problems as possible.
Want a cheatsheet that provides the essential patterns that interviewers may bring to your table.
But this series is NOT for you if you:
Want an in-depth reference that covers data structures and algorithms. (check the resources section to find great alternatives)
Looking for mathematical proofs of time and space complexities for these algorithms. (see the resource section for alternatives).
Well, Well, if you made it this far, congratulations!! It means you are in the right place!
5. RoadMap
1. General Structure
This series is written in a problem-based structure. We will discuss the most crucial algorithm problems that are well-known among Interviewers. For each problem, we will:
Explain the intuition of a brute-force solution.
Explain an optimized solution that is easy to come up with.
Discuss the time and space complexities of these solutions.
Highlight some patterns among these problems and solutions.
⚑ Note 2
I have provided brief but practical explanations for each problem in a way that targets someone who has an interview next week. Please let me know your opinion!
⚑ Note 3
Any problem in this series can be solved in multiple ways. I covered just a tiny part of those solutions, so feel free to come up with your creative ones!
2. Topics
We will cover these critical categories of problems and the algorithms that are associated with them:
Array.
Linked List.
String.
Binary Tree.
3. Prerequisites
Familiarity: You are familiar with basic data structures and algorithms. When I say familiar, I mean you should have the answers to the following questions:
What does every data structure mean in the topics list we will cover?
What is the popular way to implement each data structure?
What is Big O notation?
What is the difference between time complexity and space complexity?
What is the time complexity of each data structure’s insertion, deletion, and search operations in the topics list?
2. Python (Basics is enough!)
3. LeetCode Account: You have an account in LeetCode.
4. What do I want you to do — FGCC in Practice
Focus (F) and Group (G):
Bring paper and a pen to walk through the problems and solutions using the examples of each problem. This will help you to understand these solutions and build pattern sense.
Code these solutions back on LeetCode: This will help you to digest these patterns.
2. Convert (C) and Communicate (C)
A great way to communicate your thought process is by creating a solution template on the Leetcode website.
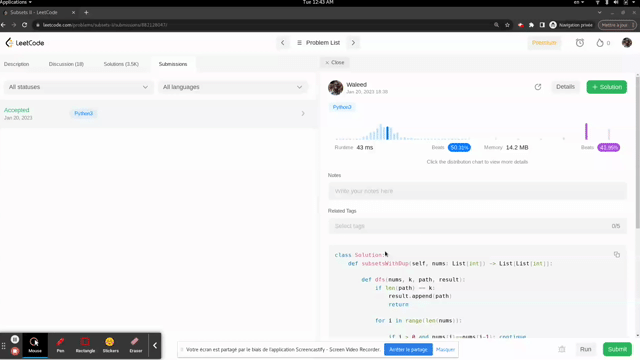
Do not wait. Share now!
6. Recap
Practicing Algorithm problems need dedication, patience, and a way to make the process enjoyable and effective. And this series comes to serve both enjoyment and effectiveness goals.
I hope this guide will be the secret weapon you will get back to every time you have a coding interview or studying algorithms, so best of luck, and let us get started!
7. Next Post
Imagine that you are playing with your friends the “Taste it and guess” game. So, instead of guessing the food name that you blindly eat, you must give one or more ingredients of this mystery food. Now, the question will be:
How can you quickly guess the names of these ingredients before dumping that bucket of ice-cold water on your head?
Later, we will discuss one of the most asked algorithms questions in coding interviews, KSum Family Problems. STAY TUNED!
New to this series?
New to the “Unfold Algorithm Coding Interviews For Data Scientists” series? Here is the full list [link to the series list]
Thank you for your reading!
You can learn more about the date of future posts for this series through my newsletter, where I talk about Algorithms, Machine Learning, and Data Science.
If you have any other questions or comments, please do not hesitate to share them with me through Newsletter | Medium | LinkedIn | Twitter. See you soon!